To Enable Drag and Drop:
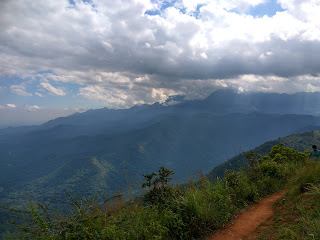
Example 1: Mouse Functionality program
Example 2: Drag and Drop Program
- Chrome Browser go to the Url: chrome://flags/
- Firefox Browser go to the Url: about:config
The mouse down sets the color of the text to red. The mouse up sets the color of the text to green.
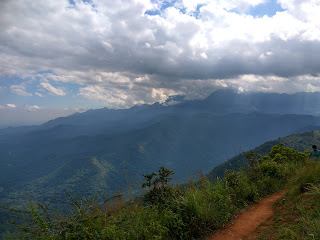
Scrolling examle: Whenever you scroll your mouse then the color of the text is changed to blue.
Drag and Drop
Drag the image back and forth between the two div elements.Example 1: Mouse Functionality program
package com.automation.tests;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.AfterTest;
import org.testng.annotations.Test;
public class MouseOperations {
WebDriver driver;
@Test
public void mouseOperations() throws InterruptedException {
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
driver.get("http://anish-selenium.blogspot.in/p/mouse-operations-in-selenium-webdriver.html");
Actions actions = new Actions(driver);
// Click a Button using WebElement
WebElement element = driver.findElement(By.id("b1"));
element.click();
Thread.sleep(5000);
// Click using Actions class object
actions.click(element).perform();
Thread.sleep(5000);
// for double click is possible only using Actions class
element = driver.findElement(By.id("b2"));
actions.doubleClick(element).perform();
Thread.sleep(5000);
// Click and Hold operations
element = driver.findElement(By.id("p1"));
actions.clickAndHold(element).perform();
Thread.sleep(4000);
actions.release(element).perform();
Thread.sleep(5000);
// Move your mouse to an WebElement
element = driver.findElement(By.id("img1"));
actions.moveToElement(element).build().perform();
Thread.sleep(5000);
// Right click on an Element
element = driver.findElement(By.id("b2"));
actions.contextClick(element).perform();
}
@AfterTest
public void afterTest() throws InterruptedException {
Thread.sleep(10000);
driver.close();
}
}
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.AfterTest;
import org.testng.annotations.Test;
public class MouseOperations {
WebDriver driver;
@Test
public void mouseOperations() throws InterruptedException {
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
driver.get("http://anish-selenium.blogspot.in/p/mouse-operations-in-selenium-webdriver.html");
Actions actions = new Actions(driver);
// Click a Button using WebElement
WebElement element = driver.findElement(By.id("b1"));
element.click();
Thread.sleep(5000);
// Click using Actions class object
actions.click(element).perform();
Thread.sleep(5000);
// for double click is possible only using Actions class
element = driver.findElement(By.id("b2"));
actions.doubleClick(element).perform();
Thread.sleep(5000);
// Click and Hold operations
element = driver.findElement(By.id("p1"));
actions.clickAndHold(element).perform();
Thread.sleep(4000);
actions.release(element).perform();
Thread.sleep(5000);
// Move your mouse to an WebElement
element = driver.findElement(By.id("img1"));
actions.moveToElement(element).build().perform();
Thread.sleep(5000);
// Right click on an Element
element = driver.findElement(By.id("b2"));
actions.contextClick(element).perform();
}
@AfterTest
public void afterTest() throws InterruptedException {
Thread.sleep(10000);
driver.close();
}
}
Example 2: Drag and Drop Program
package com.automation.tests;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class DragAndDropProgram {
public static void main(String[] args) throws Exception {
// Initiate browser
WebDriver driver = new ChromeDriver();
// maximize browser
driver.manage().window().maximize();
// Open webpage
driver.get("http://jqueryui.com/resources/demos/droppable/default.html");
// Add 5 seconds wait
Thread.sleep(5000);
// Create object of actions class
Actions act = new Actions(driver);
// find element which we need to drag
WebElement drag = driver.findElement(By.xpath(".//*[@id='draggable']"));
// find element which we need to drop
WebElement drop = driver.findElement(By.xpath(".//*[@id='droppable']"));
// this will drag element to destination
act.clickAndHold(drag).perform();
act.release(drop).perform();
//act.dragAndDrop(drag, drop).build().perform();
}
}
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class DragAndDropProgram {
public static void main(String[] args) throws Exception {
// Initiate browser
WebDriver driver = new ChromeDriver();
// maximize browser
driver.manage().window().maximize();
// Open webpage
driver.get("http://jqueryui.com/resources/demos/droppable/default.html");
// Add 5 seconds wait
Thread.sleep(5000);
// Create object of actions class
Actions act = new Actions(driver);
// find element which we need to drag
WebElement drag = driver.findElement(By.xpath(".//*[@id='draggable']"));
// find element which we need to drop
WebElement drop = driver.findElement(By.xpath(".//*[@id='droppable']"));
// this will drag element to destination
act.clickAndHold(drag).perform();
act.release(drop).perform();
//act.dragAndDrop(drag, drop).build().perform();
}
}
No comments:
Post a Comment